In Python, the repr() function plays a crucial role in representing objects as strings. It helps developers understand how an object is structured, making debugging and logging easier. But what exactly does repr() do, and how does it differ from str()? This article will break it down in simple terms, ensuring that even a 10-year-old can understand it.
What Is repr() in Python?
The repr() function in Python is used to get a string representation of an object. This representation is meant to be unambiguous, meaning it should clearly show the actual structure of the object.
For example, if you print an object using repr(), you often get a result that looks like valid Python code. This can be very useful when debugging, as it allows you to see the precise details of an object rather than just a user-friendly output.
Here’s a simple example:
python
CopyEdit
x = “Hello, world!”
print(repr(x))
Output:
python
CopyEdit
‘Hello, world!’
Notice how the output includes the quotation marks? That’s because repr() returns a representation of the string that can be used to recreate the object.
Why Do We Use repr() in Python?
The main reason we use repr() is for debugging and logging. When working with complex objects, it’s important to know their exact structure. The repr() function provides a precise representation, unlike str(), which focuses on readability.
For example, when you’re dealing with special characters in strings, repr() ensures that they are displayed correctly.
python
CopyEdit
text = “Hello\nWorld”
print(str(text))
print(repr(text))
Output:
nginx
CopyEdit
Hello
World
‘Hello\nWorld’
Here, str(text) prints the string as a human would read it, whereas repr(text) shows its exact structure, including the \n newline character.
How Is repr() Different from str()?
At first glance, repr() and str() might seem similar, but they serve different purposes.
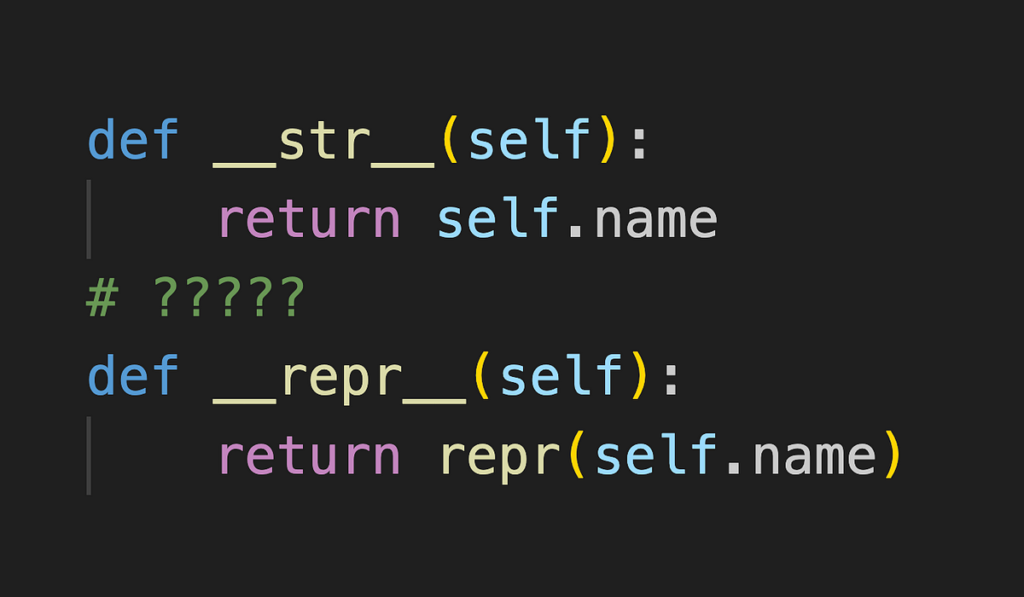
- str() is used for creating a readable string what does repr do in python of an object.
- repr() is used for creating an exact, unambiguous string representation.
repr() vs str() – Key Differences
Featurerepr()str()
Purpose For developers (debugging, logging) For end-users (displaying output)
Output Unambiguous, often valid Python code Readable, meant for humans
Special Characters Shown with escape sequences Displayed normally
Customization Can be overridden with __repr__() Can be overridden with __str__()
Example: How repr() Works in Python?
To understand better, let’s compare repr() and str() in action:
python
CopyEdit
import datetime
today = datetime.datetime.now()
print(str(today)) # User-friendly output
print(repr(today)) # Debugging-friendly output
Output:
css
CopyEdit
2025-02-24 14:30:00
datetime.datetime(2025, 2, 24, 14, 30, 0)
Here, repr(today) gives a string that can be used to recreate the object, while str(today) just presents the date and time in a readable way.
When Should You Use repr()?
You should use repr() when:
- You need a detailed, exact representation of an object.
- You’re debugging and need to see the precise structure of data.
- You want to log data in a format that can be recreated later.
On the other hand, if you’re just displaying something to the user, str() is usually the better choice.
Does repr() Work with All Data Types?
Yes! The repr() function works with almost all data types in Python, including strings, numbers, lists, tuples, dictionaries, and even custom objects.
For built-in types, Python already provides default representations. But if you define your own class, you can customize how repr() works for your objects (we’ll cover that next!).
How to Define a Custom repr() in Python?
If you’re working with your own classes, you can customize how repr() represents objects by defining the __repr__() method.
Here’s an example:
python
CopyEdit
class Car:
def __init__(self, brand, model):
self.brand = brand
self.model = model
def __repr__(self):
return f”Car(‘{self.brand}’, ‘{self.model}’)”
car = Car(“Tesla”, “Model S”)
print(repr(car))
Output:
bash
CopyEdit
Car(‘Tesla’, ‘Model S’)
Now, whenever you call repr(car), it will return a structured representation of the object.
Why Learn repr()?
Understanding repr() is important because it helps you debug effectively, write clean logs, and create maintainable code. Since repr() gives precise object details, it can save time when working with complex data structures.
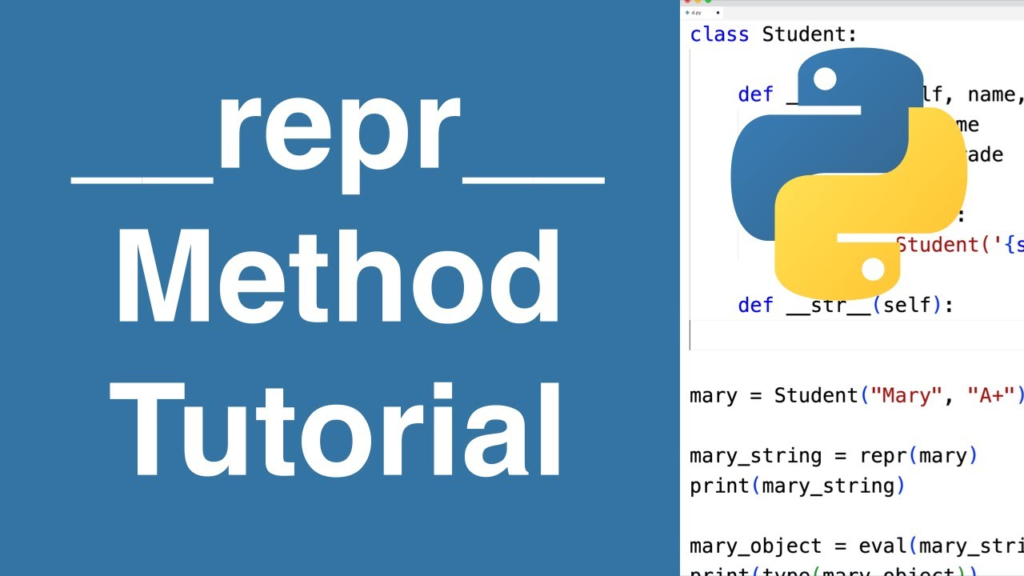
Using repr() with Strings
If you use repr() with strings, it preserves special characters and quotes:
python
CopyEdit
s = “Python’s awesome!”
print(repr(s))
Output:
arduino
CopyEdit
“Python’s awesome!”
Using repr() with Numbers
For numbers, repr() behaves similarly to str(), but it’s useful for logging exact values:
python
CopyEdit
num = 42.345
print(repr(num))
Output:
CopyEdit
42.345
Why Do We Use repr() in Python?
Developers use repr() to:
- Debug complex objects
- Store object representations in logs
- Ensure data can be recreated later
Using repr() with Lists
Lists also have a useful repr() representation:
python
CopyEdit
lst = [1, “hello”, 3.14]
print(repr(lst))
Output:
css
CopyEdit
[1, ‘hello’, 3.14]
This format makes it easy to recreate the list exactly as it was.
The Bottom Line
The repr() function in Python is a powerful tool for debugging and logging. This article will explore repr()
in detail, covering its purpose, differences from str()
, examples, and when to use it.